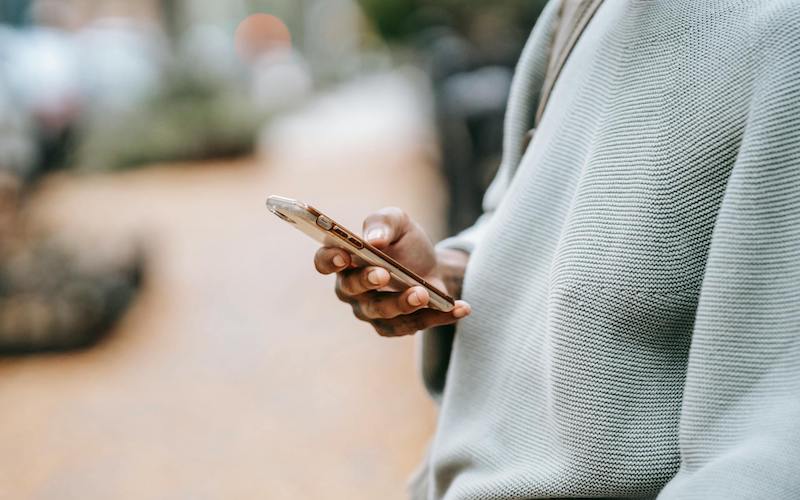
In a world of mobile technology and the internet, Real-time chat applications are the core of apps that aim to provide collaborative communication. However, implementing such functionality only makes sense if it is designed and developed considering user experience and ease of use as the priority.
If you are building a Flutter app with chat functionality, finding a feature-rich chat API is crucial. And if you are considering Stream Chat as your API solution then this blog is for you.
The blog will guide you through the steps of implementing Stream chat API in the Flutter application, along with that, you can explore the key features of the API, core functionalities of the chat application, and advanced features you can implement later in the app.
The Key Advantages of Stream Chat API
Flutter development has a great chance to benefit from Stream Chat because of the following features:
- Scalability: Keeping your user base growing and also keeping it performant is possible because Stream Chat’s infrastructure can handle large numbers of messages and users.
- Rich Features: Stream chat starts with the one-on-one, group chatting capability and message sending with timestamps as well as typing indicators among others. In addition, there are advanced functionalities that include rich media support, reactions, and threaded messages.
- Ease of Use: When using Flutter projects, IT developers find it easy to incorporate chat functionality in their application due to having a well-documented API by Stream Chat with pre-built UI components.
Chat functionalities you can build using Stream Chat Flutter
Stream Chat Flutter allows you to create a sound structure for core chat functions in your mobile app. Below are some things that can be done:
1. User Management and Authentication:
Although Stream Chat cannot directly handle user authentication, it seamlessly integrates with existing authentication solutions in a Flutter application. It is possible to use libraries like Firebase Authentication or personalized means to handle logging in and getting user-related information.
This will help Stream identify users on channels and display their details such as usernames and profile pictures.
2. Channel Management:
- Create Channels: Using the Channel class, programmatically create one-on-one or group chat channels. The channel type (type: ChannelType.messaging) should be specified together with an ID that is unique.
Dart
final channel = Channel(
id: ‘user1_user2’, // Replace with unique ID for one-on-one chat
type: ChannelType.messaging,
members: [User(id: ‘user1’), User(id: ‘user2’)], // Add members for group chat
);
- Joining and Leaving Channels: Users can join existing channels or be added by other members. Stream Chat provides methods like Channel.watch and Channel.join to manage user participation within channels.
3. Sending and Receiving Text Messages:
Users can specify the message content and other information such as timestamps and additional information of the sender. This can be done using a Message class that represents the individual message.
Dart
final message = Message(
text: ‘Hello, how are you?’,
user: User(id: ‘user1’), // Specify the sender
);
await StreamChatMessage.sendMessage(message: message, channel: channel);
The Stream Chat API allows you to automatically deliver messages to other participants in the group or channel. The new messages sent are displayed using the StreamMessageList widget, it also provides communication history.
4. Typing Indicators:
The Stream Chat has built-in functionality that displays typing indicators to other users within a channel. This helps to improve the user experience by showing real-time activity of the user and improving communication flow.
5. User Presence
Alternatively, having users online/offline status sense within a channel is possible while using Stream Chat’s presence features. This is especially useful in group chats to add the user’s availability context. This can be achieved by merely indicating who is available.
6. Basic Channel Information and Metadata:
Using Stream Chat, you are in a position to define the basic channel fields like channel name and provision for the inclusion of custom fields, if necessary. These metadata can be used for varied purposes, eg, sorting channels under suitable much less volunteering some details that may not be pertinent to the particular conversation.
7. Message Delivery Receipts
Although not the paramount feature, however, Stream chat also helps in tracking the acceptance of message receipts. It then gives you an idea, of whether the message has been sent to all recipients’ devices or not. However, it doesn’t give you a hint about if the message has been read or not.
Remember: These Features are the Building Loose for the Base of a Simple Chat Application. Stream Chat has a lot of allure to the developers because it is an open platform where a large number of useful features can be integrated in order to create a truly functional and multifaceted chat tool.
Building a Powerful Chat App with Stream Chat Flutter
Let’s begin by laying the foundation for your chat app. Here’s what you’ll need to get started:
Setting Up Stream Chat in Your Flutter Project:
- First, sign up for a free Stream Chat account at https://getstream.io/.
- Secondly, obtain your API key from the Stream Chat dashboard.
- Then add the stream_chat_flutter package to your Flutter project’s pubspec.yaml file:
YAML
dependencies:
stream_chat_flutter: ^4.2.0
- Import the necessary packages and initialize the Stream Chat client in your main Dart file:
Dart
import ‘package:flutter/material.dart’;
import ‘package:stream_chat_flutter/stream_chat_flutter.dart’;
void main() async {
final client = StreamChatClient(apiKey: ‘YOUR_API_KEY’);
await client.connectUser(
User(id: ‘user_id’), // Replace with your user information
);
runApp(MyApp(client: client));
}
Designing the Chat Interface (Figma to Flutter):
Prior to start coding, it would be fairer if you could use Figma, a most certain design instrument, and make mockups as well as the visual plan of your chat interface in Figma. Figma makes it easy for members to conveniently confirm that visuals and the overall look of the app will not differ much.
Having reached your satisfaction with the app design, you can then simply transform your design in Figma to Flutter code which will help significantly in the development phase.
Implementing One-on-One and Group Chat Functionalities:
Stream Chat has pre-existing widgets for the chat option between agents and buyers as well as group chat functionalities. The widget will be the first level container for your entire chat experience including StreamChat. There is the Chat widget inside the widget which lets the user choose whether you would like to have a private chat with one person or a group chat.
Dart
class MyApp extends StatelessWidget {
final StreamChatClient client;
const MyApp({Key? key, required this.client}) : super(key: key);
@override
Widget build(BuildContext context) {
return StreamChat(
client: client,
child: MaterialApp(
home: StreamChannel(
channel: Channel(id: ‘your_channel_id’), // Replace with channel ID
child: ChatPage(),
),
),
);
}
}
Sending and Receiving Text Messages with Timestamps and Typing Indicators:
Now let’s understand how to send and receive text messages using a timestamp and typing indicators. Here we can use a MessageList widget to display the conversation history for the given channel. The Message widget this tool helps users to send and receive new messages. With this automatic clocks and text addition, the chatting gets much more pleasant.
Advanced Features
Now that you have a solid foundation, let’s explore some of Stream Chat’s advanced features to elevate your chat app:
1. Rich Media Support:
A Stream Chat user will have access to the sharing of images, videos as well as files within chats. Utilize the FileMessage widget to empower the person with the capability of sending and receiving these kinds of messages.
Dart
Widget build(BuildContext context) {
return StreamMessageInput(
onImagePressed: (image) async {
final pickedFile = await ImagePicker().pickImage(source: ImageSource.gallery);
if (pickedFile != null) {
final file = File(pickedFile.path);
await StreamChatMessage.sendMessage(
message: Message(text: ”, attachments: [FileAttachment(file: file)]),
channel: Channel(id: ‘your_channel_id’), // Replace with channel ID
);
}
},
onFilePressed: (file) async {
// Handle other file types (e.g., videos, documents)
final pickedFile = await FilePicker.platform.pickFiles(allowMultiple: false);
if (pickedFile != null) {
final file = File(pickedFile.files.single.path);
await StreamChatMessage.sendMessage(
message: Message(text: ”, attachments: [FileAttachment(file: file)]),
channel: Channel(id: ‘your_channel_id’), // Replace with channel ID
);
}
},
);
}
2. Reactions and Threaded Messages
The views and comments that will be seen in the corresponding windows with the format of the replies and the message threaded.
- Reactions: By using a Stream Chat feature, anybody can emoji only predefined messages or likes. The MessageReactions block shows the reactions to a specific message that uses a responsible form of language. Furthermore, you can let the users define their emojis, which is something that might differentiate it from other platforms and thus give it the necessary dose of uniqueness.
Dart
Widget build(BuildContext context) {
return StreamMessage(
message: message,
child: Row(
children: [
Expanded(child: Text(message.text)),
StreamMessageReactions(message: message),
],
),
);
}
- Threaded Messages: Enable the conversations to be organized by giving the possibility to reply to the messages in a symbolic order. The MessageReplies widget from Stream Chat lets users see the connection between different thoughts in the conversation.
Dart
Widget build(BuildContext context) {
return StreamMessage(
message: message,
child: Column(
children: [
Text(message.text),
StreamMessageReplies(parentMessage: message),
],
),
);
}
3. Push Notifications
Put in place push notifications to send information on incoming messages even when the app is not in use at the moment. For FCM push notifications provider we have Stream Chat integration featured as well. The implementation steps will be given to you based on which support provider you are using.
Customization and Branding
Stream Chat deals with the user experience by all means and makes sure it is holistic. The UI components of their structure can be handled to show tremendous flexibility. By using your chosen app color scheme and typography, you can customize your chat interface to match the overall appearance of your app.
- Customizing Colors and Themes: Custom theme and color them configuration is included as one of the widget features of Stream Chat to alter the color scheme of your chat UI.
Dart
StreamChat(
client: client,
theme: ThemeData(
primaryColor: Colors.blue, // Set your desired primary color
),
child: MaterialApp(…),
);
- Customizing Message Appearance: Take advantage of the messageType utility within the StreamMessage widget so you can stylize the messages (font, size, color e.t.c.).
Using the extended functionalities of Stream Chat, such as Chat Bot, API, and Server-side Adaptation, is another way to further increase interaction with customers.
Leveraging Stream Chat’s Additional Features for Enhanced Functionality
Stream Chat offers a plethora of additional features that can be integrated to cater to specific use cases:
- Channel Moderation Tools: Preserve the orderliness of your chat channels by restricting users, deleting messages, and giving rights according to their roles.
- Chatbots and Integrations: Ensure that chatbots are integrated with some third-party services, or better still construct an option in developing your own automated tasks and user experience solutions.
- Offline Messaging Capabilities: Give a chance to users for sending or receiving messages regardless of whether they are online or offline. Next, in the queueing system messages are stored and transmitted when an internet connection is back.
Conclusion
Stream Chat provides a robust and feature-rich solution for building powerful chat functionalities within your Flutter applications. Its scalability, ease of use, and wide range of features make it an ideal choice for developers of all levels.
From basic one-on-one chat to feature-packed group chats with rich media and advanced features like threaded conversations and push notifications, Stream Chat empowers you to create engaging chat experiences for your users.
We encourage you to explore Stream Chat and delve into the world of building exceptional chat experiences within your Flutter apps. Remember, a well-integrated chat functionality can significantly enhance user engagement and foster a vibrant community within your application.
You may also like: Open Source HTML5 Games